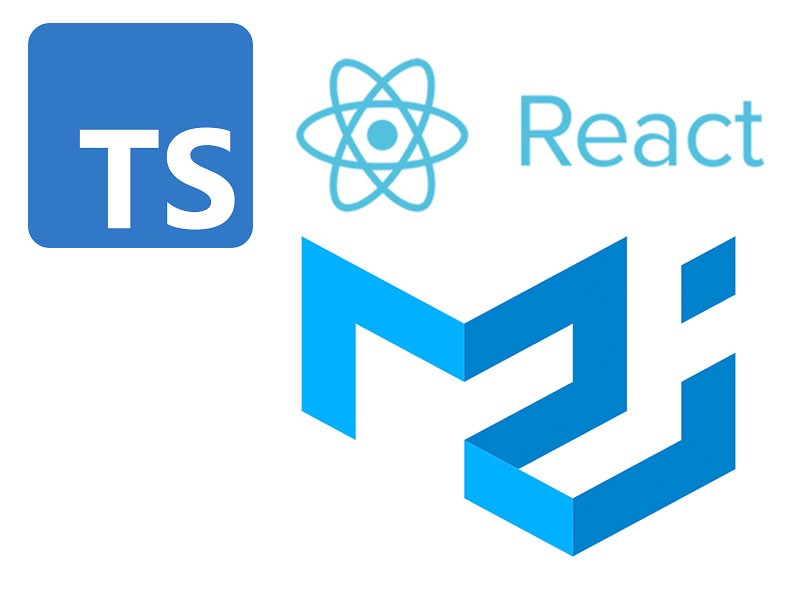
JSX Element: Understanding Its Absence of Construct or Call Signatures
When I first encountered the concept of JSX elements in React, I was puzzled by their peculiar lack of construct or call signatures. Unlike typical JavaScript classes or functions, JSX elements seemed to exist in a realm entirely of their own. Intrigued by this anomaly, I delved deeper into the subject, eagerly seeking an explanation for this unusual characteristic.
A Closer Look into JSX Elements
JSX (JavaScript XML) is a syntax extension that enables developers to write React components using a concise, XML-like syntax. Unlike regular JavaScript objects, JSX elements cannot be constructed or called using the “new” keyword or parentheses. Instead, they are created using a special syntax that resembles HTML:
const element = <div>Hello, world!</div>;
This syntax sugar allows developers to easily define the structure and content of React components. However, it begs the question: why do JSX elements lack construct or call signatures? The answer lies in their fundamental nature.
The Nature of JSX Elements
JSX elements are not true JavaScript objects; they are simply syntactic sugar that is transformed into JavaScript objects at runtime. When a JSX element is encountered during compilation, it is converted into a regular JavaScript object representing the DOM element it describes. For example, the above JSX element would be transformed into:
const element = React.createElement("div", null, "Hello, world!");
Since JSX elements are not true JavaScript objects, they cannot possess construct or call signatures. Instead, they rely on the React.createElement method to create new instances of them. This approach allows React to maintain a consistent and optimized approach to component creation and rendering.
Advantages of the Lack of Construct or Call Signatures
The absence of construct or call signatures in JSX elements offers several advantages:
- Simplicity: It simplifies the syntax for creating React components, making it easier for developers to define complex UI structures.
- Consistency: It ensures that all React components are created using the same method, promoting consistency and maintainability.
- Optimization: By relying on a single method for component creation, React can optimize the rendering process, improving performance and memory usage.
Tips for Working with JSX Elements
To effectively work with JSX elements, consider the following tips:
- Embrace the simplicity: Don’t overcomplicate the creation of JSX elements; stick to the concise and straightforward syntax.
- Use the React.createElement method directly: In certain cases, you may need to create JSX elements programmatically; use the React.createElement method for this purpose.
- Understand the limitations: JSX elements lack some features of true JavaScript objects; be aware of these limitations to avoid errors.
FAQs on JSX Elements
Q: Why do JSX elements not have construct or call signatures?
A: Because JSX elements are syntactic sugar that is transformed into JavaScript objects at runtime. They rely on the React.createElement method for creation.
Q: Can I create JSX elements using the “new” keyword or parentheses?
A: No, JSX elements must be created using the special JSX syntax or the React.createElement method.
Conclusion
The absence of construct or call signatures in JSX elements is a deliberate design choice that enhances simplicity, consistency, and optimization in React. By understanding this fundamental characteristic, developers can harness the power of JSX to create maintainable and high-performing React applications.
Are you interested in further exploring the topic of JSX elements? Let us know in the comments below!
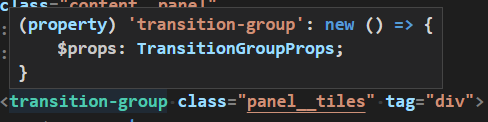
Image: github.com
Image: github.com
JSX element type does not have any construct or call signatures in v3.2.0-rc · Issue #28631 · microsoft/TypeScript · GitHub Apr 11, 2022When compiling it gave the following error: JSX element type ‘ColorButton’ does not have any construct or call signatures.ts (2604) I tried importing Button from Material UI like this: Copy. Copy. import Button from ‘@mui/material/Button’; I tried importing styled components a few different ways: Copy.